CIS 375 SOFTWARE ENGINEERING
University Of Michigan-Dearborn
Dr. Bruce Maxim, Instructor
Object Oriented Analysis Steps:
Class modeling.
(Build an object model similar to an ER diagram)
Dynamic modeling.
(Build a finite state machine type model)
Functional modeling.
(Similar to data flow diagram)
Case Study:
Elevator model.
n - elevators.
m - floors in building.
- each floor has two buttons (except ground & top).
Class Modeling
- Buttons.
- Elevators.
- Floor.
- Building.
- Movement.
- Illumination.
- Doors.
Requests.
Dynamic Modeling:
"Normal" schemas (and 1 or 2 abnormal).
-> production rules (describe state transitions).
Functional Modeling:
(Identify source & destination node)
Object-Oriented Life Cycle Model:
Fountain Model:
Bottom up design.
Class Responsibility Collaborator Model (CRC):
Responsibilities:
- Distributed system intelligence.
- State responsibility in general terms.
- Information and related behavior in same class.
- Information attributes should be localized.
- Share responsibilities among classes when appropriate.
- Collaborators build a CRC card
(Build a paper model, see if it works on paper)
On card:
Class name.
Class type.
Class characteristics.
Responsibility/collaborators.
(System is basically acted out)
O.O.D. (Booch – Abbot Method):
Define problem.
Develop process narrative for software realization of problem domain.
Formalize strategy.
- Identify object & attributes.
- Identify operations which can be applied to objects.
- Establish interfaces by showing relationships between objects and operations.
- Resolve design details to allow implementation.
- Recursively apply (2) & (3).
- Refine work done during O.O.A..
- Represent data structures associated with object attributes.
- Represent procedural derail for each operation.
Operator Classifications:
Data manipulator (add, delete, format).
Computation.
Monitors.
Basic Notation For O.O.D.:
Class diagram (static).
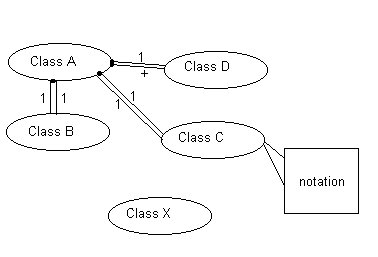
- Object diagram (dynamic).
(communication)
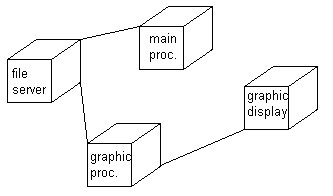
Alternative Generic Approach to O.O.D.:
- Identify the data abstraction for each sub-system.
- Identify attributes for each data abstraction.
- Identify operations for each data abstraction.
- Identify communication between objects.
- Apply inheritance where appropriate.
Object Oriented Program Design:
Undertake object oriented system requirements specification.
Identify object and their services.
Establish interactions between objects (services required & rendered).
Identification of reusable components from previous design.
Implementation of low level objects.
Introduce inheritance relationships (superclass & subclass).
Class combination and generalization.
(prototype revision – change is healthy)
Common Design Flaws:
- Classes that make direct modifications to other classes.
- Classes with too much responsibility.
- Classes with no responsibility.
- Classes with unused responsibility .
(too much junk in the class)
- Misleading names.
- Unconnected responsibility.
- Inappropriate inheritance.
- Repeated functionality.